Colorize atoms by position#
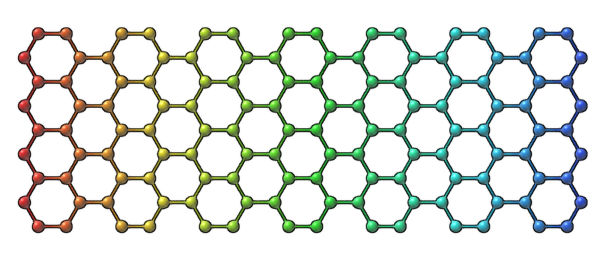
The example below demonstrates how to colorize atoms based on their position using SBColor
.
Colorize atoms based on their X coordinate#
# get all atoms
atomIndexer = SAMSON.getNodes('node.type atom')
if len(atomIndexer):
# set initial min and max values
min_x = max_x = atomIndexer[0].getX().value
# get min and max x-coordinates
for atom in atomIndexer:
x = atom.getX().value
if x > max_x: max_x = x
elif x < min_x: min_x = x
# make the operation undoable
SAMSON.beginHolding("Colorize atoms")
# colorize each atom according to its coordinate
for atom in atomIndexer:
# get RGB color for a node based on HSV color
# here we go through the hue parameter in the HSV color space to change the color
h = (atom.getX().value - min_x) / (max_x - min_x)
color = SBColor.fromHSV(239.5 / 360.0 * h, 205.0/255.0, 1.0)
# set the color of the node
atom.setColor(color)
# stop holding the undoable operation
SAMSON.endHolding()
The example below demonstrates how to colorize atoms based on their position using a color palette
.
Colorize atoms based on their X coordinate using a color palette#
# get all atoms in the active document
atomIndexer = SAMSON.getNodes('node.type atom')
if len(atomIndexer):
# set initial min and max values
min_x = max_x = atomIndexer[0].getX().value
# get min and max x-coordinates
for atom in atomIndexer:
x = atom.getX().value
if x > max_x: max_x = x
elif x < min_x: min_x = x
# create the default HSV color palette
palette = SBPalette()
# make the operation undoable
SAMSON.beginHolding("Colorize atoms")
# colorize each atom according to its coordinate
for atom in atomIndexer:
# get the color 'intensity' value, which should be in the [0, 1] range
h = 1 - (atom.getX().value - min_x) / (max_x - min_x)
# get RGB color from the palette
color = palette.getColor(h)
# set the color of the node
atom.setColor(color)
# stop holding the undoable operation
SAMSON.endHolding()