Create atoms on a sphere#
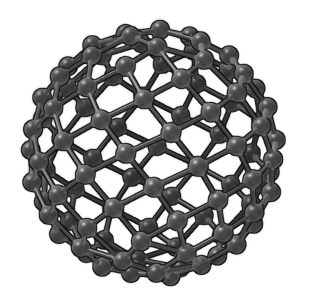
This example combines what is written in the Building section and demonstrates how to create a structural model populated with Carbon atoms placed on a sphere.
Example: create atoms on a sphere#
import math
n = 100 # number of atoms
radius = SBQuantity.angstrom(4.2) # radius of the sphere
# generate positions on a sphere
golden_ratio = (1 + math.sqrt(5)) / 2
theta = 2 * math.pi * golden_ratio
increment = 2 / n
points = []
for i in range(n):
y = ((i * increment) - 1) + (increment / 2)
r = math.sqrt(1 - pow(y, 2))
phi = (i % n) * theta
x = math.cos(phi) * r
z = math.sin(phi) * r
position = SBPosition3(radius * x, radius * y, radius * z)
points.append(position)
# create a structural model and add atoms to it
# instantiate a structural model
structuralModel = SBStructuralModel()
for i in range(n):
atom = SBAtom(SBElement.Carbon)
atom.setPosition(points[i])
structuralModel.addChild(atom)
# create covalent bonds
structuralModel.createCovalentBonds()
# make the operation undoable
SAMSON.beginHolding('Add atoms')
# hold the structural model for undo/redo
SAMSON.hold(structuralModel)
# create the structural model
structuralModel.create()
# add the structural model to the active document
SAMSON.getActiveDocument().addChild(structuralModel)
# stop the undoable operation
SAMSON.endHolding()